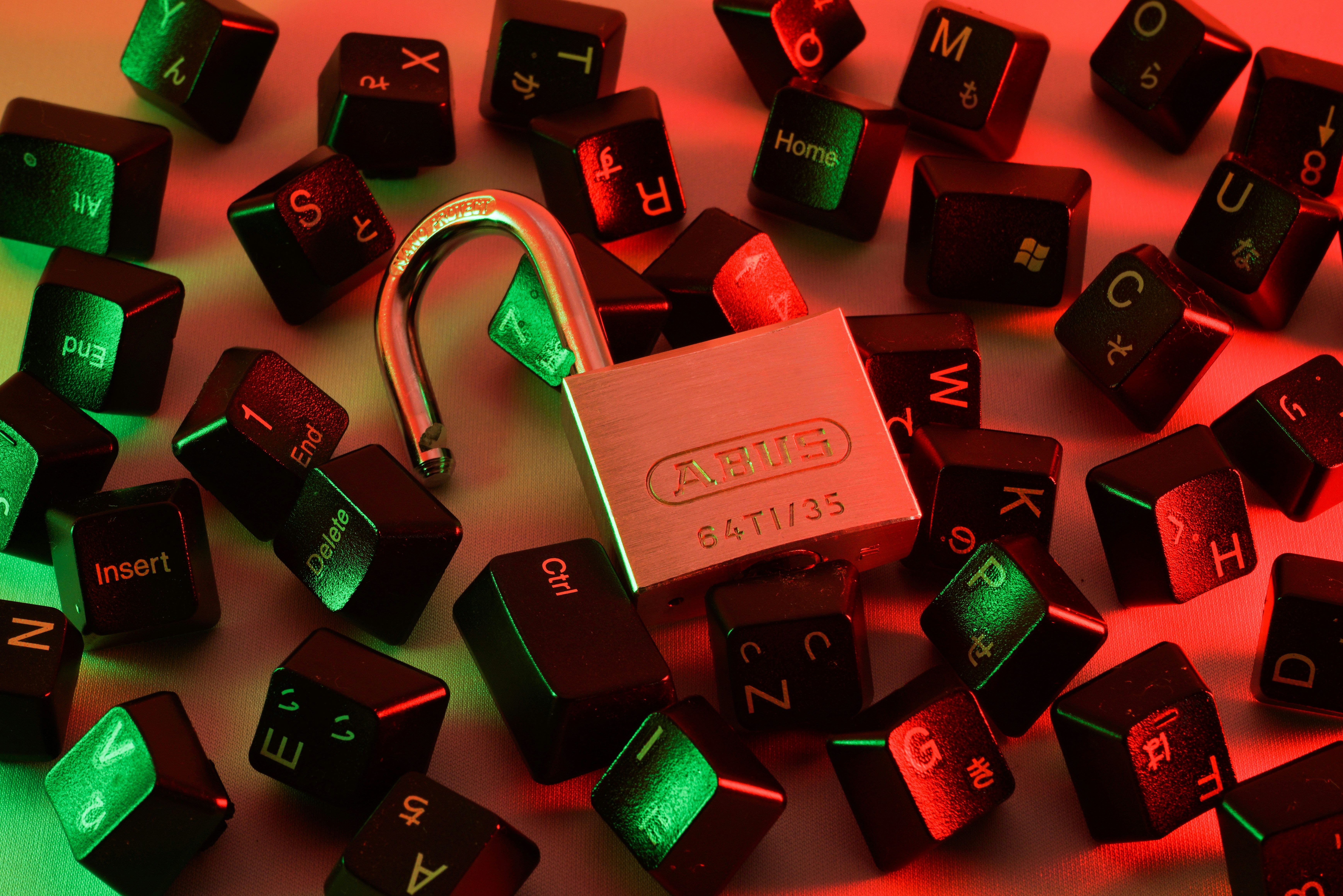
Python’s flexibility in web development, data analysis, and machine learning projects has made it one of the most popular languages in the world, but with its flexibility come many security implications. So, let’s look at five practices that can help you keep your applications more secure.
1. Validate inputs and sanitize data
Input validation and data sanitization are fundamental aspects of secure coding. It is important to validate all incoming data against the expected formats and sanitize it, as this can greatly reduce the risks posed by SQL injection and cross-site scripting (XSS) attacks. Processing un-sanitized data can have some serious implications for the safety and security of both infrastructure and customer data. It can also be used to provide attack vectors for exploits, for example, exfiltration of data, request forgeries, and injection-based attacks. For robust sanitization and validation, consider libraries like Pydantic, which can help you confirm all data incoming is what is expected. Using Pydantic, which adopts a fail-early approach, we can stop the propagation of data before it can become a problem.
2. Use secure coding libraries and tools
Leverage Python’s ecosystem of libraries and tools designed to enhance security. Here are some examples of such tools:
- Dependency Management: Use
pip-audit
to scan for vulnerabilities in dependencies. - Authentication: Implement libraries like
Flask-Login
for secure session management. - Cryptography: Protect sensitive data using the
cryptography
library for encryption andbcrypt
for hashing. - Code Analysis: Employ tools like
bandit
to automatically scan your codebase for security issues. - Hypothesis: Utilize testing libraries like
hypothesis
to help weed out edge cases and odd behaviors. By integrating these resources into your development workflow, you can greatly enhance the security of your application.
3. Keep Python and libraries up-to-date
Regularly update the Python runtime and libraries to patch vulnerabilities and maintain application security. Use modern dependency management tools like Poetry to efficiently manage and update dependencies.
Using Poetry
Start by using poetry for its strong dependency management and packaging features. Poetry has a built-in tool for tracking and updating package dependencies. To check for outdated dependencies in your project, use poetry show --outdated
. This command displays all dependencies that have newer versions. To update a specific package, use poetry update <package-name>
, or simply poetry update to upgrade all outdated dependencies. Poetry ensures that updates follow the semantic versioning constraints in your pyproject.toml
file, preserving compatibility and minimizing the risk of introducing breaking changes.
If you need help setting up poetry, check out my post here.
Using Pip
In environments without poetry, Pip is a useful tool for updating packages. Use pip list --outdated
to find outdated packages. To update a package, use pip install --upgrade <package-name>
or apply pip install --upgrade
to update multiple packages. However, for better dependency resolution and locking features, poetry is the preferred option.
Be cautious with major version updates. They may introduce breaking changes. Test your application thoroughly after updates to ensure all functionalities work as expected. This testing phase is crucial for identifying any incompatibilities or issues introduced by the updated dependencies. Address them promptly to maintain stability and security.
4. Implement error handling and logging wisely
When you’re creating your error handling and logging systems, it’s really important to think about preventing any accidental information leakage. It’s a good idea to avoid showing stack traces or any sensitive data to users. When using the logging module or bubbling up exceptions, make sure that confidential information has been sanitized. There have been a few major leaks where user passwords or banking information have been exposed in the form of system logs.
A small tip: set DEBUG = False in production, having verbose messages when developing is really helpful. But remember to turn it off in production because you don’t want to leak information regarding your environment, libraries, and so on.
5. Adhere to the principle of least privilege
It’s critical to ensure that your Python application works well with databases, file systems, and external services. You should always adhere to the principle of least privilege, which means granting only the permissions required for the job, so that the impact of a security breach is minimized. This means that you should not expose more than is necessary, not only to other parts of your system but also to your customers. It is best to draw clear lines between different parts of your system, and if there is more coupling, consider using a mediator to control the flow of data between these sections.
6. Use HTTPS for data transmission
Using HTTPS instead of HTTP is a good idea when it comes to securely transmitting data between your application and its users. This small but crucial step can effectively safeguard against possible attacks and ensure the utmost protection of crucial information, such as login credentials and personal data. Consider also having the communication between services encrypted. So it makes it harder for hackers to sniff traffic.
Tools like Let’s Encrypt, which offers free SSL/TLS certificates and allows for the consistent use of HTTPS throughout your application.
7. Conduct regular security audits and reviews
Security is a continuous process that requires regular auditing of your codebase and dependencies to identify vulnerabilities. The threat of zero-day exploits is ever present, and some of the worst leaks of data have been due to poor auditing and testing. It is also important to conduct thorough code reviews with a focus on security. It is also wise to have regular meetings with the team to discuss areas where there might be potential security vulnerabilities and to make sure everyone is following all current best practices. By taking this proactive approach, you can identify and address potential issues early on, ensuring that your application remains resilient against emerging threats.
Final thoughts
To ensure the security of Python applications, it is crucial to adopt a comprehensive strategy. This includes implementing thorough input validation, utilizing specialized libraries and tools, minimizing privileges, handling errors cautiously, and consistently conducting audits and reviews. By integrating these practices into your development process, you can enhance the security of your Python projects, thereby protecting both your data and the data of your users.